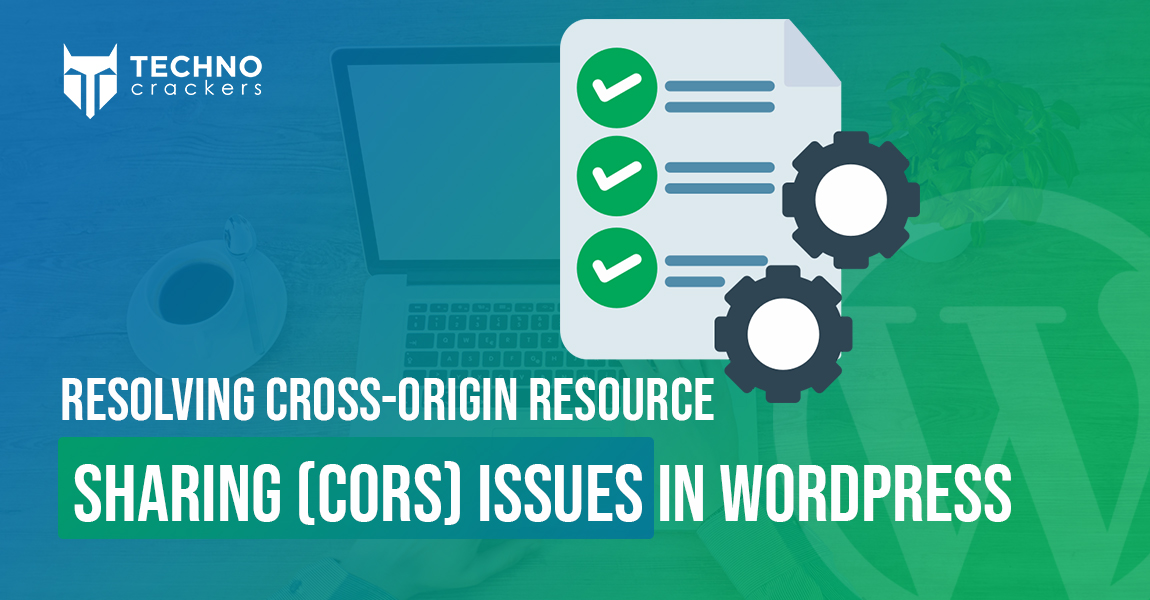
Cross-Origin Resource Sharing (CORS) is both a critical security mechanism and a source of common frustration for WordPress developers. Designed to prevent unauthorized access to resources on different domains, CORS ensures data security but can inadvertently block legitimate resources, like fonts, JavaScript files, or API responses, that your site depends on.
Imagine designing a flawless layout only to see broken fonts or encountering errors when integrating third-party APIs. These issues often arise because of restrictive CORS policies, which require specific headers to grant access between domains. This guide breaks down the problem, helping you understand why CORS issues occur and providing actionable solutions tailored to WordPress environments. Whether your site uses Apache, Nginx, or the WordPress REST API, you’ll learn how to debug, configure, and test CORS settings effectively to ensure smooth functionality without compromising security.
What is CORS and Why Does it Matter?
CORS is a browser mechanism that controls how resources on one domain are accessed by another domain. Suppose your WordPress site attempts to load external resources (e.g., fonts, scripts, or API data). In that case, the server hosting those resources must explicitly allow such access by including proper CORS headers in its response.
Common Symptoms of CORS Issues:
- Fonts not loading on your site with errors in the browser console like:Access to font at ‘https://example.com/font.woff’ from origin ‘https://yoursite.com’ has been blocked by CORS policy.
- JavaScript fetching data from an API fails with:No ‘Access-Control-Allow-Origin’ header is present on the requested resource.
Step 1: Debugging CORS Issues
Use Browser Dev Tools:
- Open your browser’s developer tools (right-click on your site and select Inspect, then go to the Console tab).
- Look for CORS-related errors (e.g., “Access to the resource has been blocked by CORS policy”).
- Identify the blocked resource and its URL from the error message.
Key Information to Note:
- The domain of the blocked resource.
- Whether the issue occurs for fonts, scripts, images, or API calls.
Step 2: Configuring CORS Headers in .htaccess
If the resource is hosted on your WordPress site, you can resolve the issue by configuring CORS headers in your .htaccess file (for Apache servers).
Example for Allowing Fonts:
Add the following lines to your .htaccess file:
<IfModule mod_headers.c> <FilesMatch "\.(ttf|ttc|otf|eot|woff|woff2|font.css)$"> Header set Access-Control-Allow-Origin "*" </FilesMatch> </IfModule>
Example for General Resources:
Allow all resources to be shared with specific domains:
<IfModule mod_headers.c> Header set Access-Control-Allow-Origin "https://example.com" Header set Access-Control-Allow-Methods "GET, POST, OPTIONS" Header set Access-Control-Allow-Headers "X-Requested-With, Content-Type, Accept" </IfModule>
- Replace https://example.com with the domain that requires access.
- Avoid using * for sensitive resources, as it allows access from any domain.
Restart Apache Server
For changes to take effect, restart your Apache server:
sudo service apache2 restart
Step 3: Configuring CORS Headers in Nginx
If you’re using Nginx as your web server, you can add CORS headers in your site’s configuration file.
Example Configuration:
location ~* \.(eot|ttf|woff|woff2|otf)$ { add_header Access-Control-Allow-Origin "*"; } location / { add_header Access-Control-Allow-Origin "https://example.com"; add_header Access-Control-Allow-Methods "GET, POST, OPTIONS"; add_header Access-Control-Allow-Headers "X-Requested-With, Content-Type, Accept"; }
- Save the changes and reload Nginx:
sudo nginx -s reload
Step 4: Adding CORS Headers to WordPress REST API Responses
If the issue occurs with custom REST API endpoints in WordPress, you can programmatically add CORS headers using the rest_api_init action.
Code Example:
Add the following code to your theme’s functions.php file or a custom plugin:
add_action('rest_api_init', function () { header("Access-Control-Allow-Origin: *"); header("Access-Control-Allow-Methods: GET, POST, OPTIONS"); header("Access-Control-Allow-Headers: X-Requested-With, Content-Type, Accept"); });
Notes:
- Replace * with a specific domain for better security.
- Use this approach only for REST API endpoints served by your WordPress site.
Step 5: Testing the Fix
- Clear your browser cache and reload your site.
- Use browser dev tools to verify that the blocked resources are now loading correctly.
- Check the response headers for the affected resource. You should see the Access-Control-Allow-Origin header in the response.
Best Practices for Handling CORS Issues
- Restrict Access: Avoid using * as the value for Access-Control-Allow-Origin. Specify only the domains that require access.
- Monitor Logs: Regularly check server logs to identify and address CORS-related issues.
- Secure APIs: For APIs, implement proper authentication mechanisms (e.g., API keys or OAuth) to complement CORS headers.
Resolving CORS issues in WordPress requires a mix of understanding and strategic implementation. You can unblock essential resources by correctly configuring CORS headers in your server’s .htaccess or Nginx configuration files—or adding headers programmatically for REST API responses—while maintaining tight security controls. Debugging tools like browser developer consoles will help pinpoint errors, ensuring targeted fixes.
Remember, best practices like restricting domains instead of using wildcards (*) and regularly monitoring server logs will safeguard your resources. With these steps, you can confidently address CORS challenges, ensuring your WordPress site runs smoothly and delivers a seamless user experience.